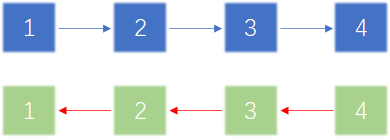
题目要求
定义一个函数,输入一个链表的头节点,反转该链表并输出反转后链表的头节点。
输入: 1->2->3->4->5->NULL
输出: 5->4->3->2->1->NULL
原题链接:剑指 Offer 24. 反转链表
解题思路
废话不多说,直接画图肝就行,遇到这种题,画个三四个节点,敲着代码,脑海里有个图解过程就会好很多。题刷多了,图画多了做题就会有感觉了~~对于指针的运用目前还不熟,但感觉如果是双指针的话,无非一个后移,然后另外一个追上这个指针,反复循环。
后期在做总结,指针部分也可参考LeetCode-删除链表中的节点(单双、指针实现)。
ps:ppt画图真的好累😫
辅助栈
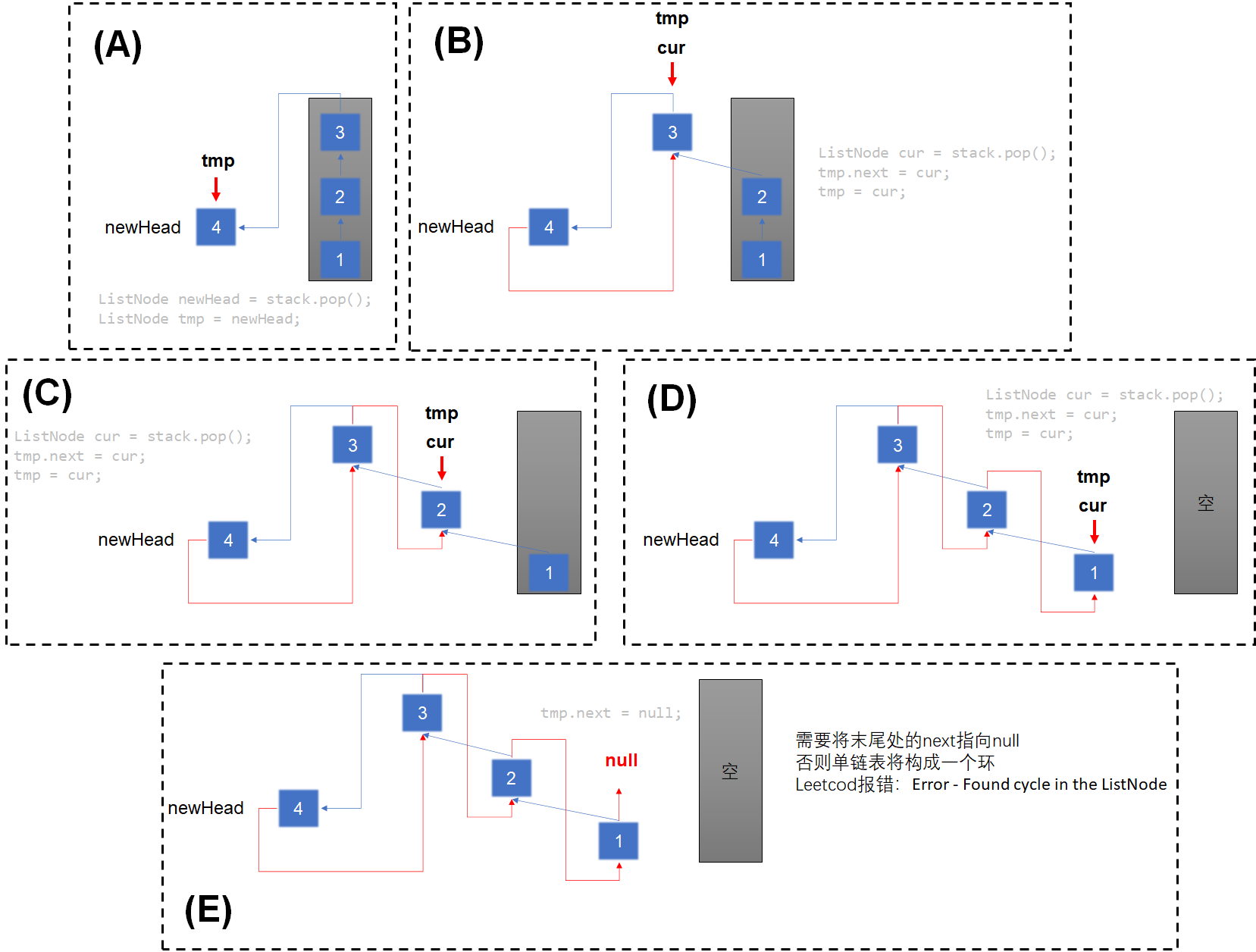
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Solution { public ListNode reverseList(ListNode head) { if(head == null || head.next == null) return head;
Stack<ListNode> stack = new Stack<>(); while(head != null){ stack.push(head); head = head.next; }
ListNode newHead = stack.pop(); ListNode tmp = newHead;
while(!stack.isEmpty()){ ListNode cur = stack.pop(); tmp.next = cur; tmp = cur; } return newHead; }
}
|
双指针
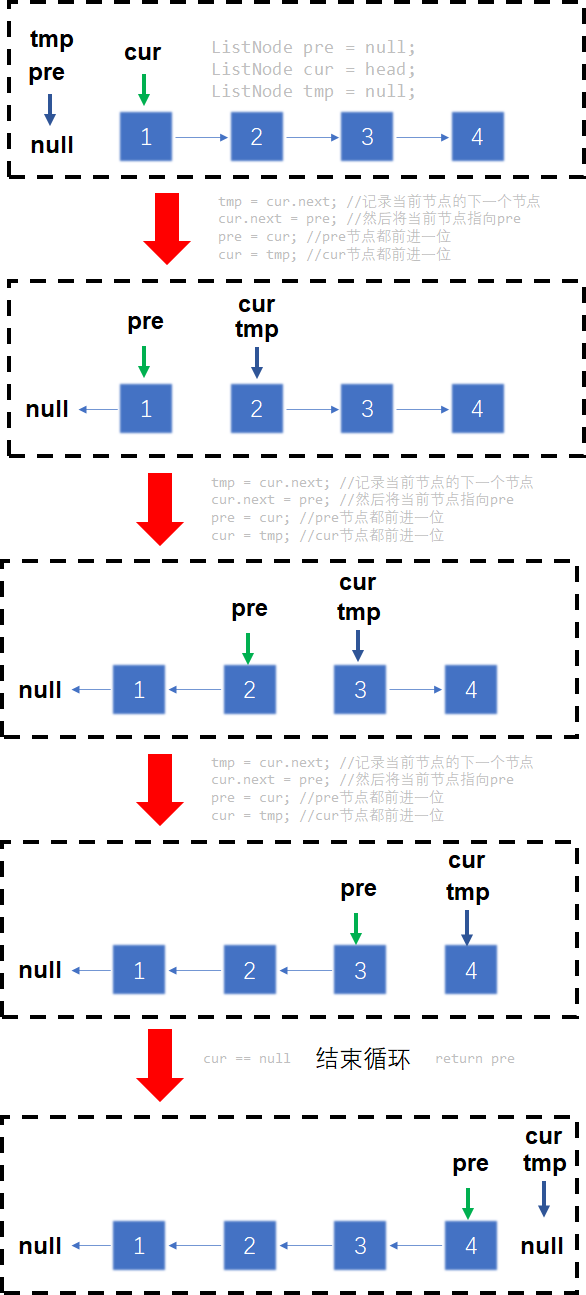
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class Solution { public ListNode reverseList(ListNode head) { ListNode pre = null; ListNode cur = head; ListNode tmp = null; while(cur!=null) { tmp = cur.next; cur.next = pre; pre = cur; cur = tmp; } return pre; } }
|
头插法
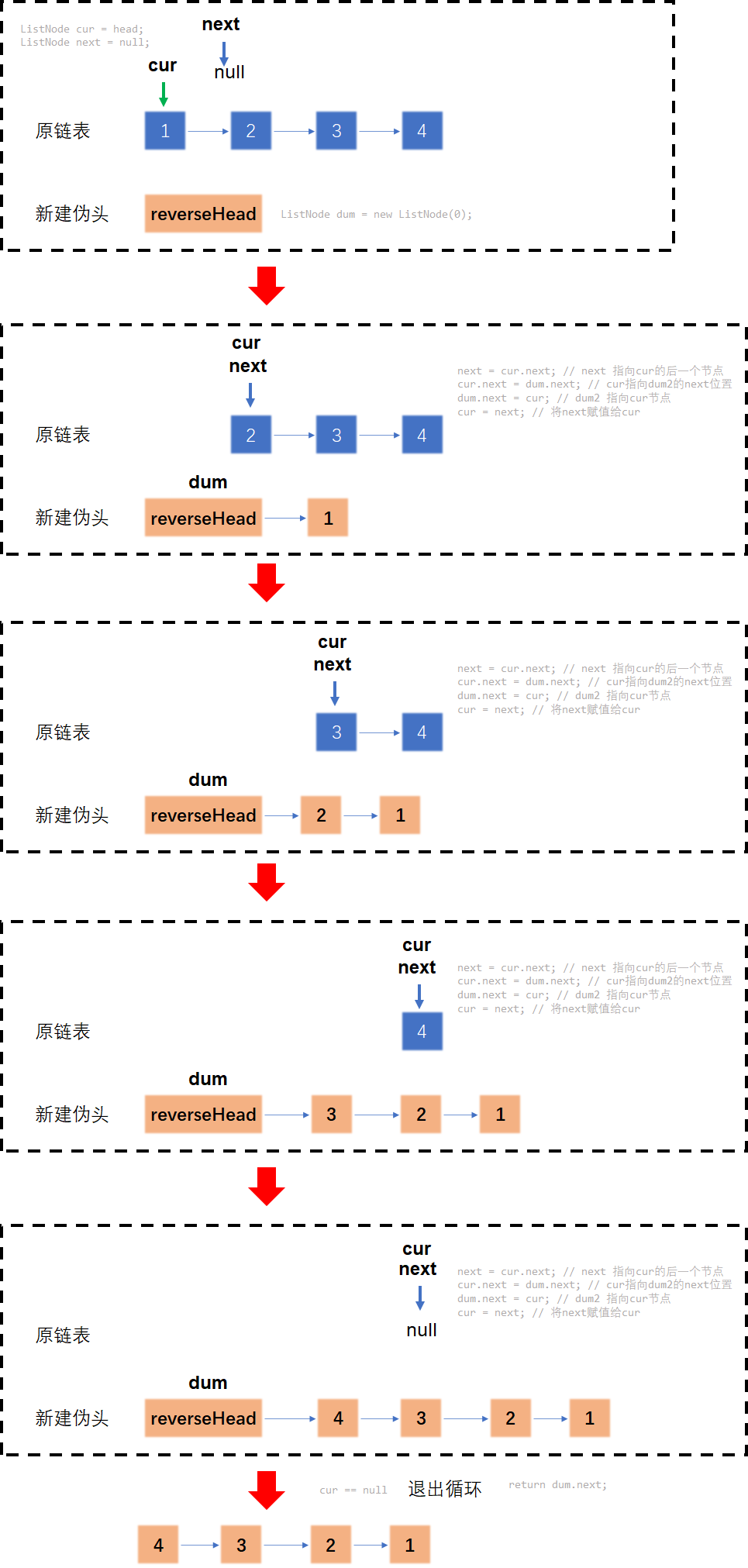
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public ListNode reverseList(ListNode head) { ListNode dum = new ListNode(0);
if(head == null || head.next == null) return head;
ListNode cur = head; ListNode next = null;
while(cur != null){ next = cur.next; cur.next = dum.next; dum.next = cur; cur = next; }
return dum.next; }
}
|