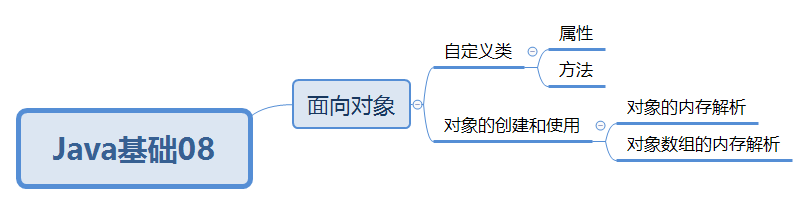
面向对象(OOP)思想
面向对象编程:OOP (Object-Oriented Programmming)
面向对象分析:OOA (Object-Oriented Analysis)
面向对象设计:OOD (Object-Oriented Design)
面向对象是一种思想,是基于面向过程而言的,就是说面向对象是将功能等通过对象来实现,将功能封装进对象之中,让对象去实现具体的细节;这种思想是将数据作为第一位,而方法或者说是算法作为其次,这是对数据一种优化,操作起来更加的方便,简化了过程。
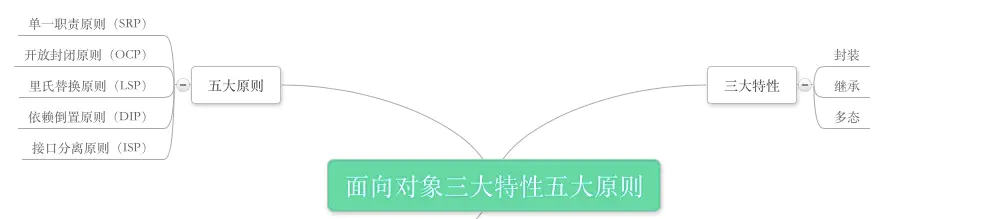
参考:
OOA/OOD/OOP细讲
什么是面向对象(OOP)
类
- 定义类(考虑修饰符、名)
- 编写类的属性 (考虑修饰符、属性 类型、属性名 、初始化值)
- 编写类的方法 (考虑修饰符、返回值类型、方法名、形参等)

简单的Person类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| package com.yuanbaoqiang.exer;
public class Test { public static void main(String[] args){ Person p1 = new Person();
p1.name = "Tom"; p1.age = 18; p1.sex = 1;
p1.study(); p1.showAge();
int newAge = p1.addAge(2); System.out.println(p1.name + "的新年龄为:" + newAge);
System.out.println(p1.age);
Person p2 = new Person(); p2.showAge();
}
}
class Person { String name; int age;
int sex;
public void study() { System.out.println("studying"); } public void showAge(){ System.out.println("age:" + age); }
public int addAge(int i){ age +=2; return age; }
}
|
对象
对象的内存解析
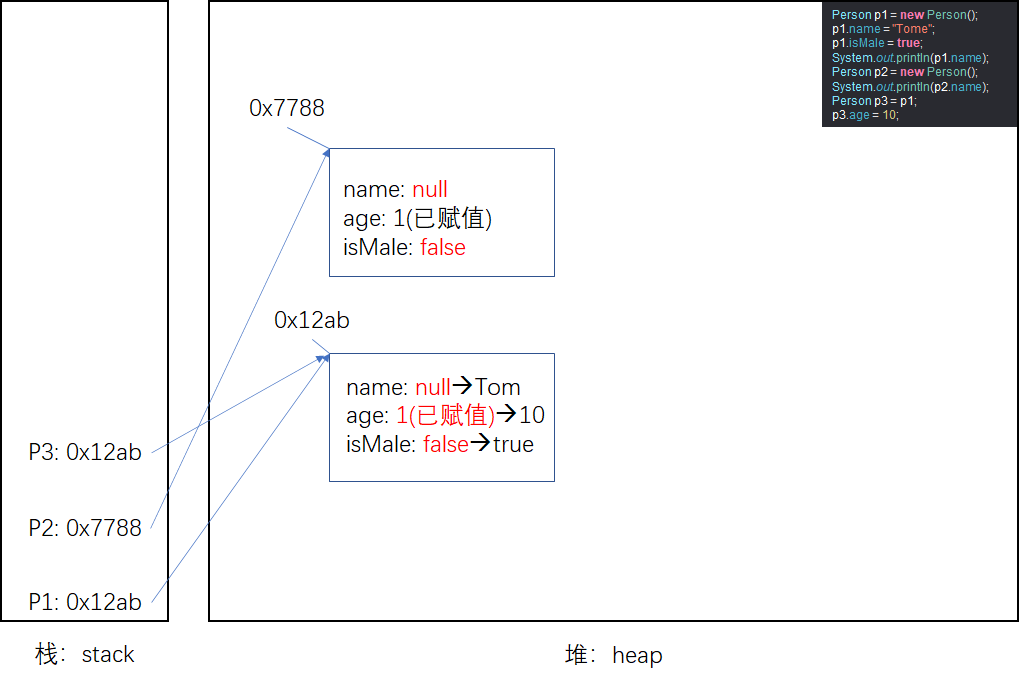
对象数组的内存解析
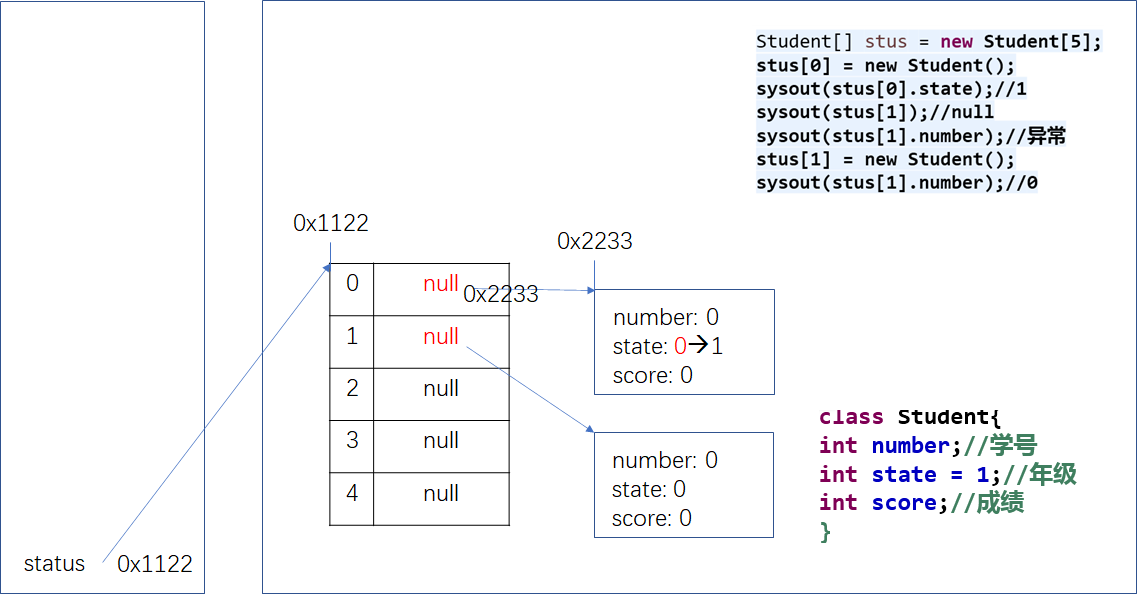
练习
定义类Student1,包含三个属性:学号number(int),年级state(int),成绩score(int)创建20个学生对象,学号1到20,年级和成绩都由随机数确定。
- 问题一:打印出3年纪(state值为3)的学生信息
- 问题二:使用冒泡顺序按照学生成绩排序,并遍历所有学生信息
可将一些功能进行封装成方法,优化代码结构。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110
|
package com.yuanbaoqiang.exer;
public class StudentAgain { public static void main(String[] args){
Student1[] s1 = new Student1[20];
for (int i = 0; i < s1.length; i++) {
s1[i] = new Student1();
s1[i].number = i + 1;
s1[i].state = (int)(Math.random() * (6 - 1 + 1) + 1);
s1[i].score = (int)(Math.random() * (100 - 0 + 1) + 0);
}
StudentAgain sa = new StudentAgain();
sa.Print(s1); System.out.println("===========================================");
sa.searchState(s1,3); System.out.println("===========================================");
sa.sort(s1); sa.Print(s1);
}
public void Print(Student1[] s1){ for (int i = 0; i < s1.length; i++) { System.out.println(s1[i].info()); } }
public void searchState(Student1[] s1, int state){ for (int i = 0; i < s1.length; i++) { if(s1[i].state == 3){ System.out.println(s1[i].info()); } } }
public void sort(Student1[] s1){ for (int i = 0; i < s1.length - 1; i++) { for (int j = 0; j < s1.length - 1 -i; j++) { if(s1[j+1].score < s1[j].score){ Student1 temp; temp = s1[j]; s1[j] = s1[j+1]; s1[j+1] = temp; } } } }
}
class Student1{ int number, state, score;
public String info(){ return "编号为 " + number + " 的学生" + ",年级为 " + state + ",分数为 " + score; } }
|